I created a project that insert on PostgreSQL table Pato through Api rest of Spring.
First i download the project from Spring Initializr
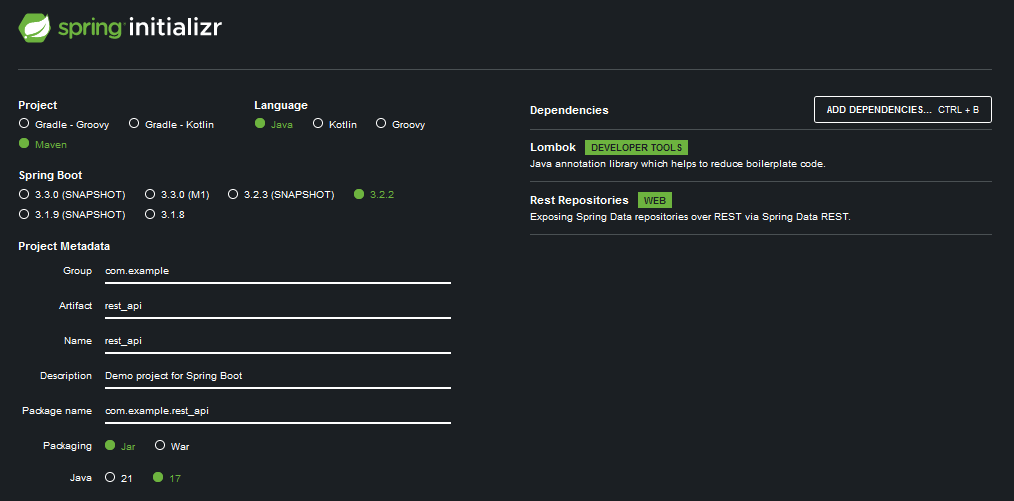
i created the java class Pato :
@Entity
public class Pato {
private Pato() {
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Integer id;
public String getNombre() {
return nombre;
}
public void setNombre(String nombre) {
this.nombre = nombre;
}
public int getEdad() {
return edad;
}
public void setEdad(int edad) {
this.edad = edad;
}
private String nombre;
private int edad;
}
Then i created PatoControler
import lombok.extern.slf4j.Slf4j;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
import java.util.ArrayList;
import java.util.List;
@RestController
@Slf4j
public class PatoControler {
PatoRepository repPato ;
public PatoControler(PatoRepository repPato) {
this.repPato = repPato;
}
@GetMapping
public String getObtenerNombre () {
return "pato";
}
@GetMapping("/patos")
public Iterable<Pato> getObtenerNombres () {
// System.out.printf("obtener nombres");
return repPato.findAll();
}
private List<Pato> patoList = new ArrayList<>();
@PostMapping("/patos")
public List<Pato> crearNombre ( @RequestBody Pato patroclo)
{
System.out.printf("crear nombres " + patroclo.getNombre());
repPato.save(patroclo);
patoList.add(patroclo);
return patoList;
}
}
after i created a interface to extends CrudRepository
import org.springframework.data.repository.CrudRepository;
import java.util.List;
public interface PatoRepository extends CrudRepository <Pato, Integer>{
List<Pato> findByNombre(String Nombre);
Pato findById(int id);
}
I had to add dependencies to pom.xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<scope>runtime</scope>
</dependency>
i had to add the connection to the database of supabase
spring.jpa.hibernate.ddl-auto=update
spring.datasource.url=jdbc:postgresql://db.mydb.supabase.co:5432/postgres
spring.datasource.username=user01
spring.datasource.password=123456
spring.jpa.properties.hibernate.dialect= org.hibernate.dialect.PostgreSQLDialect
Test with Postman
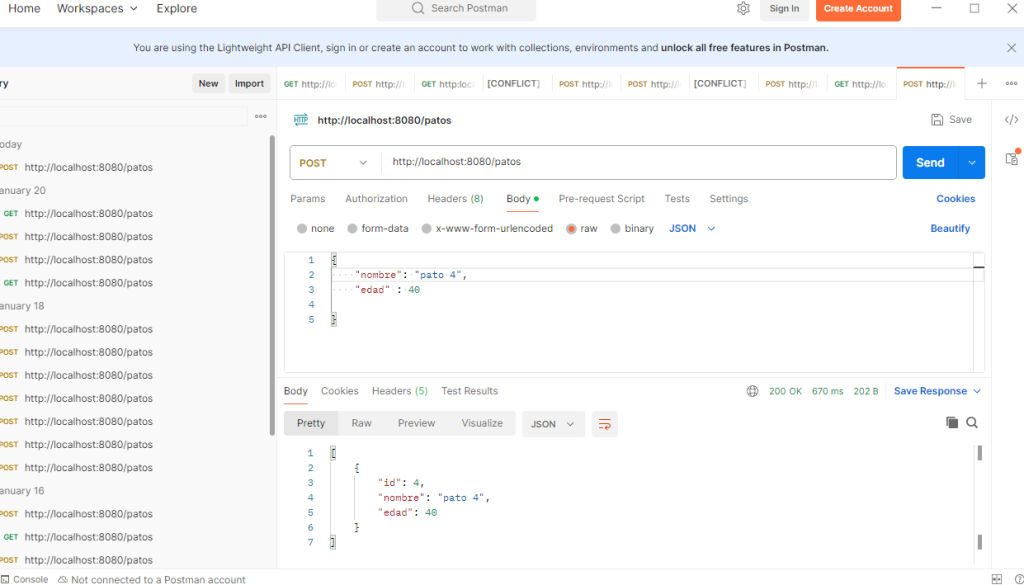
Then we see on the database on SupaBase
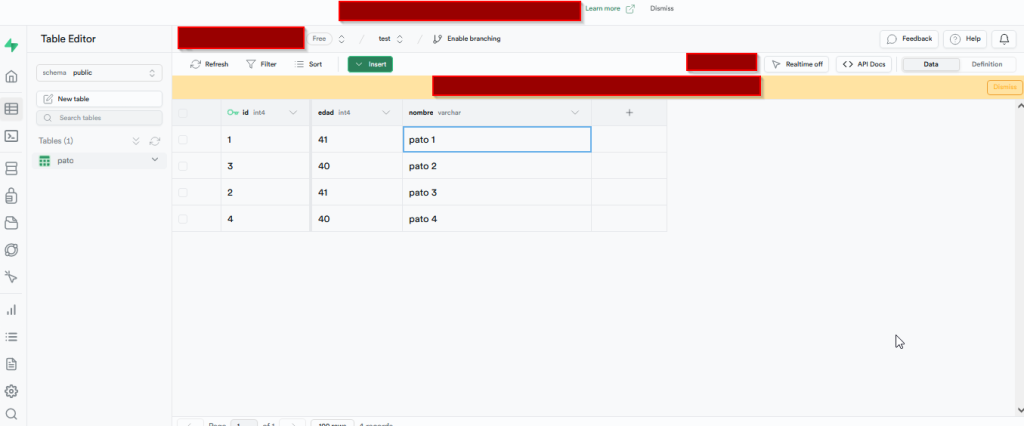
https://juliovaldiviamarin.com
Leave a Reply
You must be logged in to post a comment.